Memory, Variables and Edge Detection:
The purpose of this circuit is to read input from a switch and illuminate each of the 5 LEDs in a particular sequence based on the number of times the switch is pressed. Thus, the first time the switch is pressed the LED to the far right is illuminated. This sequence is repeated each time the switch is pressed until the 5th LED is illuminated. The fifth time the switch is pressed a buzzer sounds and all five LEDs are turned off. The user can then repeat this process. The circuit contains one switch with a 10kOhm pull down resistor going to ground. The switch is connected to pin 12 and the 5VDC power supply.
The circuit contains one switch with a 10kOhm pull down resistor going to ground. The switch is connected to pin 12 and the 5VDC power supply.
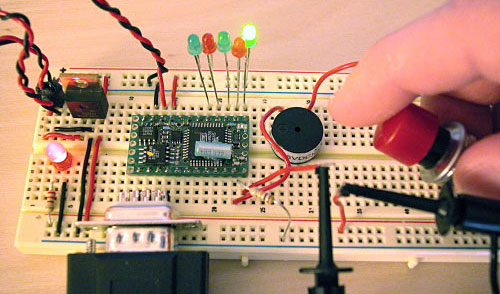
How it works:
The circuit reads from pin 12 (nowState = getPin(12)) the number of times the switch is pressed. Each time the switch is pressed, we increment switchCount. We use switchCount + 12 (12 is just an offset related to the pin location on the BX-24) to set high pins 13-17 in a sequence. The LEDs remain lit until switchCount is greater than five. On the fifth press the piezo buzzer sounds, and the LEDs are turned off.
This is an important exercise beacuse the goal is to keep track of the number of times the switch is pressed without continously incrementing the counter. Because the switch can be held closed indefinitely, it is imprortant to increment switchCount only once per press. To do this we must keep track of the current state and previous state of the switch. deltaState tells us when there is a change in the button's state, and is often referred to as edge detection. However, we want to increment switchCount only when the button is closed. We use the if (nowState = 1) and (deltaState = 1) then evaluation to check if the button is closed and if there is a difference in the previous state as compared to the current state. The code is shown below:
dim switchCount as byte
dim previousState as byte
dim deltaState as byteSub
main()
call delay(0.5) 'start program with a half-second delay
dim nowState as byte
switchCount = 0
call putpin(5,0) 'make sure pin 5 is low
previousState = 0
do
nowState = getPin(12)
'check to see if there was a difference between
'the current State and the previous state
deltaState = abs(nowState - previousState)
if (nowState = 1) and (deltaState = 1) then
switchCount = switchCount + 1
'increment the switch count
debug.print cStr(switchCount)
'illuminate LEDs on pins 13 - 17
call putPin(switchCount + 12,1)
'if the count switch is greater than 5
'exit the do loop and recall the main program
if switchCount = 5 then
call putpin(11,1)
delay(0.2)
call putpin(11,0)
exit do
end if
previousState = 1
elseif (nowState = 0) then
previousState = 0
end if
loop
'reset the pins low and call the main program again
dim i as byte
for i = 13 to 17
call putPin(i,0)
next
'jump to the beginning of the main subroutine
call main()
end sub
|